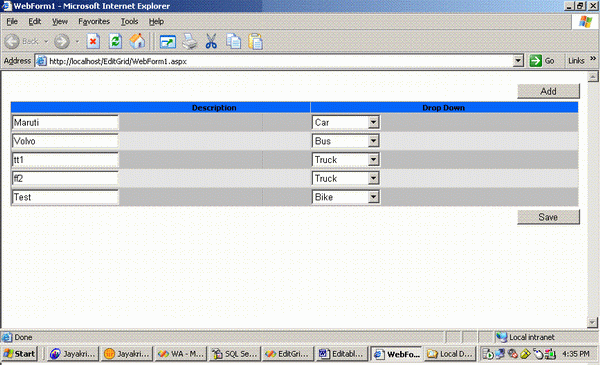
Introduction
This article explains how to make an ASP.NET editable DataGrid
.
Using the code
This a web application with a virtual directory named as EditGrid. You may create the same virtual directory to run this application by VS.NET 2003, or create any other virtual directory and map the path to this directory to access it from the browser. As I have used Access 2002 as my database, you need to have MS Access installed on your machine.
Important Sections of this application
DataGrid
with TextBox
and DataGrid
as item templates for enable editing, and two buttons, one for adding a new row to the grid and another for saving the grid data to the database.
<table class="TABLE_MAIN_NEW" width="100%" ID="Table1">
<tr vAlign="top">
<td colSpan="4"></td>
<td align="right"><asp:button id="btnAdd" runat="server"
CausesValidation="False" Text="Add" Width="91px" Height="22"
CssClass="BUTTON_MEDIUM"></asp:button>
</td>
</tr>
<tr vAlign="top">
<td align="center" colSpan="5">
<div class="dgrStyle_new">
<asp:datagrid id="dgEditGrid" runat="server"
CssClass="DGR_MAIN" AutoGenerateColumns="False" width="100%">
<AlternatingItemStyle CssClass="DGR_ALTERNATE"></AlternatingItemStyle>
<ItemStyle CssClass="DGR_ITEM"></ItemStyle>
<HeaderStyle CssClass="DGR_HEADER"></HeaderStyle>
<Columns>
<asp:TemplateColumn HeaderText="Description">
<ItemTemplate>
<asp:TextBox MaxLength="50" runat="server"
ID="txtDESCRIPTION" cssclass="TEXTBOX_MEDIUM_DG1"
Text='<%#Container.DataItem("DESCRIPTION")%>' />
</ItemTemplate>
</asp:TemplateColumn>
<asp:TemplateColumn HeaderText="Drop Down">
<ItemTemplate>
<asp:DropDownList id="ddlDrop" runat="server"
Width="100px" cssclass="DROPDOWN_SMALL_DG"></asp:DropDownList>
</ItemTemplate>
</asp:TemplateColumn>
<asp:BoundColumn DataField="itemNumber" HeaderText="Item_No"
Visible="False"></asp:BoundColumn>
</Columns>
</asp:datagrid>
</div>
</td>
</tr>
<tr vAlign="top">
<td colSpan="4"></td>
<td align="right">
<asp:button id="btnSave" runat="server"
CausesValidation="False" Text="Save" Width="91px" Height="22"
CssClass="BUTTON_MEDIUM">
</asp:button>
</td>
</tr>
</table>
- Load routine for loading data to a
DataSet
from the database.
Private Sub LoadFile()
Dim con As OleDbConnection
Dim dtaAdapter As OleDbDataAdapter
Dim dstCopy As New DataSet
Try
Dim strPath As String = Server.MapPath("Data") & "\Auto.mdb"
con = New System.Data.OleDb.OleDbConnection("provider=" & _
"Microsoft.Jet.OLEDB.4.0; " & "data source=" & strPath)
dtaAdapter = New OleDbDataAdapter("Select * from Table1", con)
dtaAdapter.Fill(dstCopy, "tbl_Temp_Data")
con.Close()
Session.Add("TempData", dstCopy)
dgEditGrid.DataSource = dstCopy.Tables(0)
dgEditGrid.DataBind()
Catch ex As Exception
Throw ex
End Try
End Sub
BindDrop
routine for loading data from DataSet
to grid.
Private Sub BindDrop()
If Not IsNothing(Session("TempData")) Then
dstTempData = Session("TempData")
If Not IsNothing(dstTempData.Tables(0)) _
AndAlso dstTempData.Tables(0).Rows.Count > 0 Then
dgEditGrid.DataSource = dstTempData.Tables(0)
dgEditGrid.DataBind()
End If
End If
End Sub
- Add button
Click
event for adding a new row to the DataSet
, there by adding a new row to the grid.
Private Sub btnAdd_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnAdd.Click
Try
If Not IsNothing(Session("TempData")) Then
dstTempData = Session("TempData")
Dim drwRow As DataRow
Dim intPreRow As Integer
intPreRow = dstTempData.Tables(0).Rows.Count
Dim dgItem As DataGridItem
For Each dgItem In dgEditGrid.Items
Dim intRow As Integer = dgItem.ItemIndex
If intRow <> -1 Then
Dim txtDescription As TextBox = _
CType(dgItem.Cells(0).FindControl("txtDESCRIPTION"), _
TextBox)
Dim ddlDGDrop As DropDownList = _
CType(dgItem.Cells(1).FindControl("ddlDrop"), _
DropDownList)
dstTempData.Tables(0).Rows(intRow)("DESCRIPTION")_
= txtDescription.Text
dstTempData.Tables(0).Rows(intRow)("Type")_
= ddlDGDrop.SelectedValue
End If
Next
Dim intFinalRow As Integer
drwRow = dstTempData.Tables(0).NewRow()
dstTempData.Tables(0).Rows.Add(drwRow)
intFinalRow = dstTempData.Tables(0).Rows.Count - 1
dstTempData.Tables(0).Rows(intFinalRow)("DESCRIPTION") = ""
dstTempData.Tables(0).Rows(intFinalRow)("Type") = ""
Session("TempData") = dstTempData
BindDrop()
End If
Catch objException As Exception
Finally
End Try
End Sub
- Save button
Click
event for saving data from grid to the database.
Private Sub btnSave_Click(ByVal sender As Object, _
ByVal e As System.EventArgs) Handles btnSave.Click
Dim con As OleDbConnection
Dim dtaAdapter As OleDbDataAdapter
Dim dstCopy As New DataSet
Dim cmdGrid As OleDbCommand
Dim strQuery As String
Dim intError As Integer = 0
Dim strPath As String = Server.MapPath("Data") & "\Auto.mdb"
con = New System.Data.OleDb.OleDbConnection("provider" & _
"=Microsoft.Jet.OLEDB.4.0; " & "data source=" & strPath)
con.Open()
Dim dgItem As DataGridItem
For Each dgItem In dgEditGrid.Items
Dim txtDesc As TextBox = _
CType(dgItem.Cells(0).FindControl("txtDESCRIPTION"), TextBox)
Dim ddlType As DropDownList = _
CType(dgItem.Cells(1).FindControl("ddlDrop"), DropDownList)
Dim item As Integer
If txtDesc.Text.Trim <> String.Empty And _
ddlType.SelectedItem.Text <> "-Select-" Then
If IsNumeric(dgItem.Cells(2).Text) Then
item = CInt(dgItem.Cells(2).Text)
strQuery = "Update Table1 SET DESCRIPTION='" + txtDesc.Text _
+ "',TYPE='" + ddlType.SelectedItem.Text + _
"' WHERE itemNumber=" + CStr(item)
Else
item = 0
strQuery = "INSERT INTO Table1(DESCRIPTION,TYPE) VALUES('" _
+ txtDesc.Text + "','" + _
ddlType.SelectedItem.Text + "')"
End If
cmdGrid = New OleDbCommand(strQuery, con)
cmdGrid.ExecuteNonQuery()
strQuery = String.Empty
cmdGrid.Dispose()
End If
Next
con.Close()
LoadFile()
End Sub
BindListControls
routine for populating data in the dropdown list.
Private Sub BindListControls(ByRef ddlDrop As DropDownList)
Dim lstType As ListItem
lstType = New ListItem("-Select-", "-Select-")
ddlDrop.Items.Insert(0, lstType)
lstType = New ListItem("Car", "Car")
ddlDrop.Items.Insert(1, lstType)
lstType = New ListItem("Bus", "Bus")
ddlDrop.Items.Insert(2, lstType)
lstType = New ListItem("Truck", "Truck")
ddlDrop.Items.Insert(3, lstType)
lstType = New ListItem("Bike", "Bike")
ddlDrop.Items.Insert(4, lstType)
End Sub
DataGrid ItemDataBound
event for populating dropdownlists.
Private Sub dgEditGrid_ItemDataBound(ByVal sender As Object, _
ByVal e As System.Web.UI.WebControls.DataGridItemEventArgs) _
Handles dgEditGrid.ItemDataBound
Try
If e.Item.ItemType = ListItemType.Item Or e.Item.ItemType_
= ListItemType.AlternatingItem Then
Dim intRow As Integer = e.Item.ItemIndex
If intRow = -1 Then
Exit Sub
End If
If Not IsNothing(Session("TempData")) Then
dstTempData = Session("TempData")
Dim CurrentDrop As String = _
dstTempData.Tables(0).Rows(intRow)("Type")
Dim itemSub As ListItem
Dim ddlDGDrop As DropDownList = _
CType(e.Item.Cells(1).FindControl("ddlDrop"), DropDownList)
BindListControls(ddlDGDrop)
itemSub = ddlDGDrop.Items.FindByText(CurrentDrop)
If Not itemSub Is Nothing Then itemSub.Selected = True
End If
End If
Catch objException As Exception
Finally
End Try
End Sub
Points of Interest
I found out this method while converting a Lotus Notes application to Windows.
Jeykey is a software engineer working for an MNC and he has been to programming from 1999.
His programming experience includes Dot net, Java, VB 6.0 SQL AND ASP. He is into playing chess, football, cricket and badminton.He is interested in human psychology and farming.